API management
This section provides an overview of the requests (methods) used in the Fabric Services System REST API and provides basic examples.
Methods
The method defines the operation with a resource. The following methods are used in the Fabric Services System API:
- GET
- Retrieves a resource or set of resources
- POST
- Creates a resource or set of resources
- PUT
- Updates or replaces an existing resource or set of resources
- PATCH
- Update one or more specific properties of an existing resource or set of resources
- DELETE
- Removes a resource or a set of resources
Working with the Swagger UI
The Swagger UI describes each service (resource) in the API.
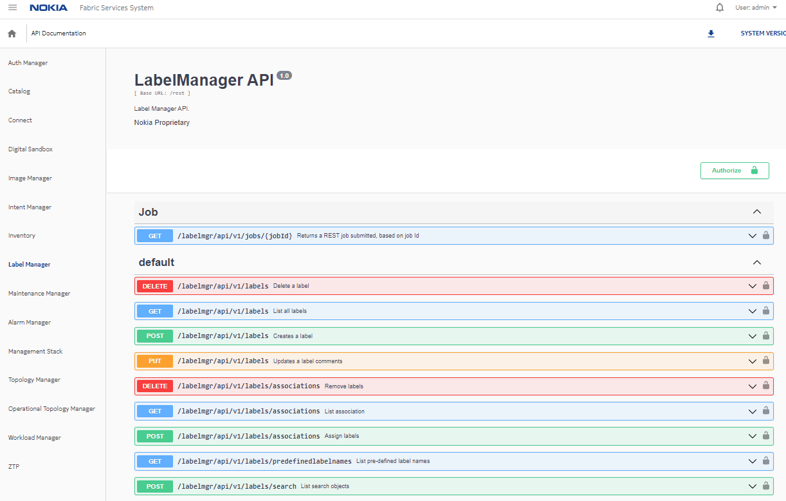
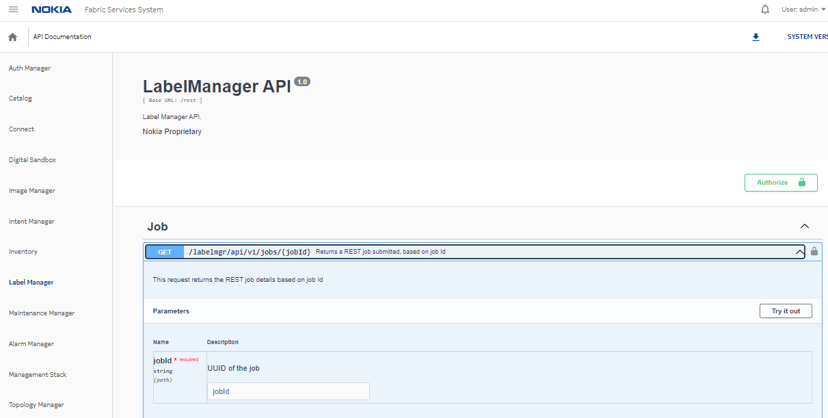
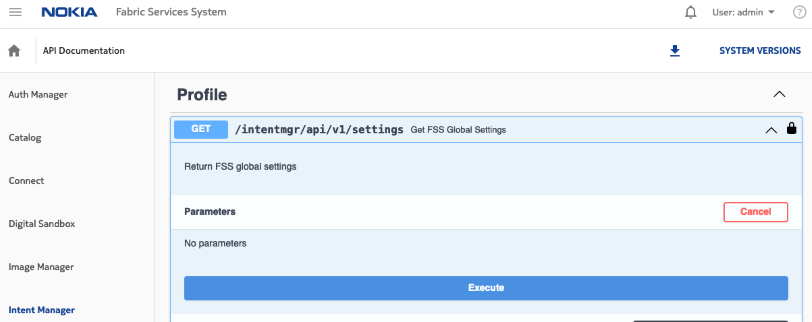
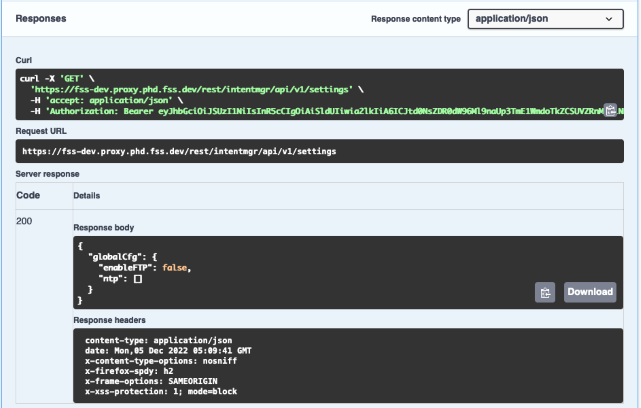
Retrieving a resource
Use a GET
request to retrieve an object or group of objects. When you
use the UUID, the request returns a single object in JSON format; if you do not use a UUID,
the request returns a JSON list of objects.
Retrieve a list of fabric objects
curl --fail -s -X GET -H "Authorization: Bearer <ACCESS TOKEN>" -H "Content-Type: application/json; charset=utf-8" "https://fss.domain.tld/rest/intentmgr/api/v1/intents"
[
{
...
"fabric": [
{
"deployInfo": {
"isFailed": false,
"reason": "Delete Workload workload 1 Deployment Done.",
"timestamp": "2022-11-22T19:19:12Z",
"transactionId": "435561924323704832"
},
"isDigitalSandbox": false,
"name": "edge1_realnet_fabric",
"state": "DeploymentDone",
"uuid": "434325057192460288",
"version": "1.0"
}
],
...
"uuid": "434325057192329216",
"version": "1.0",
"wlCount": 0
},
{
...
"fabric": [
{
"deployInfo": {
"isFailed": false,
"reason": "Workload APP01 Deployment Done",
"timestamp": "2022-11-22T22:58:46Z",
"transactionId": "435584022265987072"
},
"isDigitalSandbox": true,
"name": "DSPOD01_gatenet_fabric",
"state": "DeploymentDone",
"uuid": "435562509898940416",
"version": "1.0"
}
],
...
"uuid": "435562509865320448",
"version": "1.0",
"wlCount": 0
}
]
Retrieve a single fabric with its UUID
curl --fail -s -X GET -H "Authorization: Bearer <ACCESS TOKEN>" -H "Content-Type: application/json; charset=utf-8" "https://fss.domain.tld/rest/intentmgr/api/v1/intents/434325057192329216"
{
...
"fabric": [
{
"deployInfo": {
"isFailed": false,
"reason": "Delete Workload workload 1 Deployment Done.",
"timestamp": "2022-11-22T19:19:12Z",
"transactionId": "435561924323704832"
},
"isDigitalSandbox": false,
"name": "edge1_realnet_fabric",
"state": "DeploymentDone",
"uuid": "434325057192460288",
"version": "1.0"
}
],
...
"uuid": "434325057192329216",
"version": "1.0",
"wlCount": 0
}
Create a resource
Use a POST
request to create a new resource or set of resources. When
you create a new object, you must provide a JSON object that contains all the attributes
of the object as defined in the API specifications.
Create a label
curl -X 'POST' \
'https://fss.domain.tld/rest/labelmgr/api/v1/labels' \
-H 'accept: application/json' \
-H 'Authorization: Bearer <ACCESS TOKEN>' \
-H 'Content-Type: application/json' \
-d '{
"comments": "",
"defaultLabel": false,
"externalID": "",
"name": "Edge-Link",
"value": "edgelabel"
}'
{
"externalID": "",
"name": "Edge-Link",
"value": "edgelabel",
"comments": "",
"defaultLabel": false
}
Updating an object
Use a PUT
request to update an existing object.
Update a label
This PUT
request updates the comments for a label
curl -X 'PUT' \
'https://fss.domain.tld/rest/labelmgr/api/v1/labels' \
-H 'accept: application/json' \
-H 'Authorization: Bearer <ACCESS TOKEN>' \
-H 'Content-Type: application/json' \
-d '{
"externalID": "",
"name": "Edge-Link",
"value": "edgelabel",
"comments": "Updated Comment",
"defaultLabel": false
}'
{
"externalID": "",
"name": "Edge-Link",
"value": "edgelabel",
"comments": "Updated Comment",
"defaultLabel": false
}
Deleting an object
You use a DELETE
request to remove an object or a set of objects.
DELETE requests
The followingDELETE
request removes minor severity
alarms:curl -X 'DELETE' \
'https://fss.domain.tld/rest/alarmmgr/api/v1/alarms?severity=minor' \
-H 'accept: application/json' -H 'Authorization: Bearer <ACCESS TOKEN>'
The
following DELETE
request removes an alarm with a UUID
424197038574403584:curl -X 'DELETE' \
'https://fss.domain.tld/rest/alarmmgr/api/v1/alarms/424197038574403584' \
-H 'accept: application/json' -H 'Authorization: Bearer <ACCESS TOKEN>'
Bulk API operations
A bulk API request is a single HTTPS request that contains multiple objects instead of a
single object. For example, a regular POST
requests contains the
definition of a single object and creates a single object, and then returns the created
object. A bulk POST
request contains a list of objects (of the same
type and with the same hierarchy) where each object is handled individually and created;
the return contains a list of created objects instead of a single object.
Bulk API requests use the same URIs as for single object requests. If single object is received, it handles it as regular API call. If a list of objects is received, it handles it as a bulk API request.
Bulk API requests are supported for POST
, PUT
and
DELETE
bulk API calls.
Limitations
The maximum number of objects allowed for each bulk operation is as follows:
Operation | Number of objects |
---|---|
POST | 200 |
PUT | 200 |
DELETE | 150 |
Method | Endpoint | Details |
---|---|---|
POST/DELETE | /auth/api/{version}/users/:uuid/roles | add or remove role from user |
POST/DELETE | /auth/api/{version}/users/:uuid/usergroups | add or remove users from user-group |
POST/DELETE | /auth/api/{version}/usergroups/:uuid/users | associate or disassociate user from user-group |
POST | /intentmgr/api/{version}/intents/:uuid/fabrics/:fabricid/accept | accept, commit, or update diff |
POST and PUT requests
All POST
and PUT
endpoints that accept the JSON body
(object) to create or update an object also expose bulk POST
and bulk
PUT
endpoints.
Create a single region
POST http://fss.domain.tld/rest/intentmgr/api/v1/regions
{ /* single region object*/ }
Create multiple regions
POST http://fss.domain.tld/rest/intentmgr/api/v1/regions
[ { /* region1 object*/ }, { /* region2 object*/ }, { /* region3 object*/ } ]
Delete requests
DELETE
endpoints that accept resource ID in the URI or accept the JSON
object as body (as identifier) also expose bulk DELETE
endpoints.
Delete devices identified by UUID
The following example deletes a single device by its UUIDs:DELETE http://fss.domain.tld/rest/inventory/api/v1/devices/{uuid}
The following example deletes multiple devices identified by their
UUIDs:DELETE http://fss.domain.tld/rest/inventory/api/v1/devices?uuid=1234&uuid=5678&uuid=4534
Delete objects by their labels
The following example deletes a single label identified by its name and value:DELETE http://fss.domain.tld/rest/labelmgr/api/v1/labels
{ "name": "Tenant", "value": "tenant1" }
DELETE http://fss.domain.tld/rest/labelmgr/api/v1/labels
[ { "name": "Tenant", "value": "tenant1" }, { "name": "tenant", "value": "tenant2" }, { "name": "tenant", "value": "tenant3" } ]
Bulk API response
A bulk API request can include the following response codes:
Code | Description |
---|---|
200 |
All actions were successful; information for each object is provided in the body. |
207 |
Not all actions were successful; check the body for each object to identify the errors. |
4XX |
Something is wrong with the entire bulk API request and it cannot be handled. This code is returned when incorrect formatting is used or when too many objects have been provided. |
Response structure
The response structure allows for the easy handling of the response for each separate object; each object has a unique response. You can use the index in the response to map the response back to each object in the original request. The response also includes the overall status that lists how many actions were successful and how many failed.
{
"response": [
{
"status": $STATUS_CODE_FIRST_OBJECT,
"data": $RETURN_DATA_FIRST_OBJECT
},
{
"status": $STATUS_CODE_SECOND_OBJECT,
"data": $RETURN_DATA_SECOND_OBJECT
}
],
"responseMetadata": {
"success": $NUMBER_OF_SUCCESSFUL_OBJECTS,
"failure": $NUMBER_OF_FAILED_OBJECTS,
"total": $TOTAL_NUMBER_OF_OBJECTS
}
}
Variable | Description |
---|---|
$STATUS_CODE_*_OBJECT |
Provides the HTTP response code that would be returned if a single object API request had been made with the specified action. For possible values, see Response codes. |
$RETURN_DATA_*_OBJECT |
Provides the HTTP response data that would be returned if a single object API request had been made with the specified action. For instance, it contains the full objects data if the creation went fine or an error output if something went wrong for the specific object. |
$NUMBER_OF_SUCCESSFUL_OBJECTS |
Specifies for how many objects the action was successful. |
$NUMBER_OF_FAILED_OBJECTS |
Specifies for how many objects the action failed. |
$TOTAL_NUMBER_OF_OBJECTS |
Specifies the total number of objects provided. This value is
the sum of $NUMBER_OF_SUCCESSFUL_OBJECTS and
$NUMBER_OF_FAILED_OBJECTS . |